The C Programming Language Wikipedia
Table Of Content
Multi-character constants (e.g. 'xy') are valid, although rarely useful — they let one store several characters in an integer (e.g. 4 ASCII characters can fit in a 32-bit integer, 8 in a 64-bit one). Since the order in which the characters are packed into an int is not specified (left to the implementation to define), portable use of multi-character constants is difficult. Variables declared within a block by default have automatic storage, as do those explicitly declared with the auto[note 2] or register storage class specifiers. The auto and register specifiers may only be used within functions and function argument declarations; as such, the auto specifier is always redundant. Objects declared outside of all blocks and those explicitly declared with the static storage class specifier have static storage duration. Some compilers warn if an object with enumerated type is assigned a value that is not one of its constants.
White House urges developers to dump C and C++
However, it is also possible to allocate a block of memory (of arbitrary size) at run-time, using the standard library's malloc function, and treat it as an array. The first line of the program contains a preprocessing directive, indicated by #include. This causes the compiler to replace that line with the entire text of the stdio.h standard header, which contains declarations for standard input and output functions such as printf and scanf. Most of the recently reserved words begin with an underscore followed by a capital letter, because identifiers of that form were previously reserved by the C standard for use only by implementations. Since existing program source code should not have been using these identifiers, it would not be affected when C implementations started supporting these extensions to the programming language.
Jump statements
Conversely, it is possible for memory to be freed, but is referenced subsequently, leading to unpredictable results. Typically, the failure symptoms appear in a portion of the program unrelated to the code that causes the error, making it difficult to diagnose the failure. Such issues are ameliorated in languages with automatic garbage collection. At the same time, C rules for the use of arrays in expressions cause the value of a in the call to setArray to be converted to a pointer to the first element of array a. Thus, in fact this is still an example of pass-by-value, with the caveat that it is the address of the first element of the array being passed by value, not the contents of the array.
Data types
The C library functions, including the ISO C standard ones, are widely used by programs, and are regarded as if they were not only an implementation of something in the C language, but also de facto part of the operating system interface. Unix-like operating systems generally cannot function if the C library is erased. This is true for applications which are dynamically as opposed to statically linked.
Daniel C. Dennett, Widely Read and Fiercely Debated Philosopher, 82, Dies - The New York Times
Daniel C. Dennett, Widely Read and Fiercely Debated Philosopher, 82, Dies.
Posted: Mon, 22 Apr 2024 07:00:00 GMT [source]
Arithmetic operators
The members of a structure are stored in consecutive locations in memory, although the compiler is allowed to insert padding between or after members (but not before the first member) for efficiency or as padding required for proper alignment by the target architecture. The size of a structure is equal to the sum of the sizes of its members, plus the size of the padding. In general, the widths and representation scheme implemented for any given platform are chosen based on the machine architecture, with some consideration given to the ease of importing source code developed for other platforms.
The C Programming Language
The formatting of these operators means that their precedence level is unimportant. Appendix A, the reference manual, is not the standard, but our attempt to convey the essentials of the standard in a smaller space. It is meant for easy comprehension by programmers, but not as a definition for compiler writers—that role properly belongs to the standard itself. C's usual arithmetic conversions allow for efficient code to be generated, but can sometimes produce unexpected results.
Strings
To illustrate this, consider an array a declared as having 10 elements; the first element would be a[0] and the last element would be a[9]. The precedence table determines the order of binding in chained expressions, when it is not expressly specified by parentheses. All assignment expressions exist in C and C++ and can be overloaded in C++. When not overloaded, for the operators &&, ||, and , (the comma operator), there is a sequence point after the evaluation of the first operand. Contemporary C compilers include checks which may generate warnings to help identify many potential bugs.
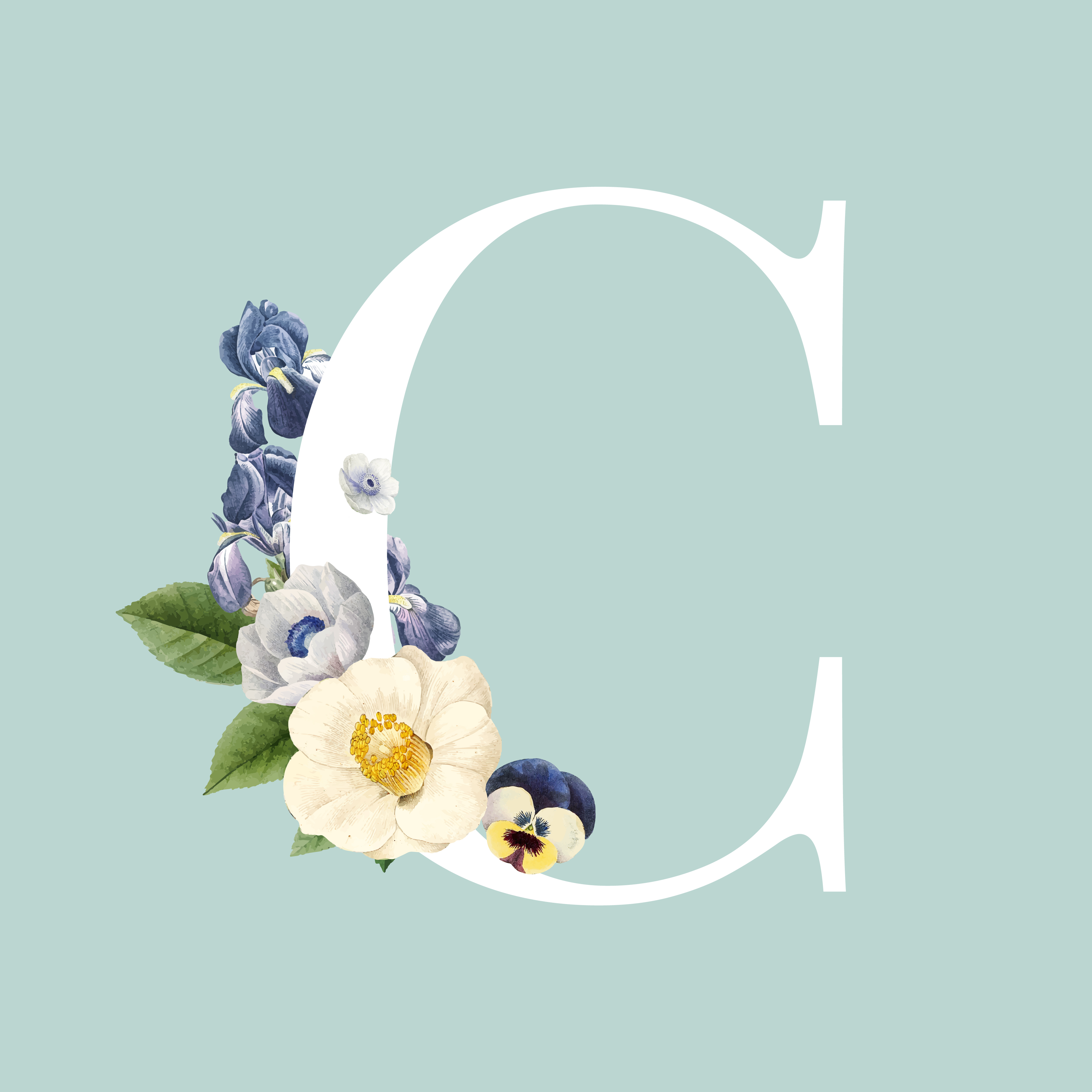
When used outside of all blocks, it indicates that the storage has been defined outside of the compilation unit. The extern storage class specifier is redundant when used on a function declaration. It indicates that the declared function has been defined outside of the compilation unit. The standard header file float.h defines the minimum and maximum values of the implementation's floating-point types float, double, and long double. It also defines other limits that are relevant to the processing of floating-point numbers. This declares the enum colors type; the int constants RED (whose value is 0), GREEN (whose value is one greater than RED, 1), BLUE (whose value is the given value, 5), and YELLOW (whose value is one greater than BLUE, 6); and the enum colors variable paint_color.
GCC 13 compiler collection backs C++ 23, Go 1.18
If this is not done, the variable becomes a dangling pointer which can lead to a use-after-free bug. However, if the pointer is a local variable, setting it to NULL does not prevent the program from using other copies of the pointer. Local use-after-free bugs are usually easy for static analyzers to recognize. Therefore, this approach is less useful for local pointers and it is more often used with pointers stored in long-living structs.

A common alternative to wchar_t is to use a variable-width encoding, whereby a logical character may extend over multiple positions of the string. Variable-width strings may be encoded into literals verbatim, at the risk of confusing the compiler, or using numerical backslash escapes (e.g. "\xc3\xa9" for "é" in UTF-8). The UTF-8 encoding was specifically designed (under Plan 9) for compatibility with the standard library string functions; supporting features of the encoding include a lack of embedded nulls, no valid interpretations for subsequences, and trivial resynchronisation. Encodings lacking these features are likely to prove incompatible with the standard library functions; encoding-aware string functions are often used in such cases. C's integer types come in different fixed sizes, capable of representing various ranges of numbers. The type char occupies exactly one byte (the smallest addressable storage unit), which is typically 8 bits wide.
Assigning values to individual members of structures and unions is syntactically identical to assigning values to any other object. The only difference is that the lvalue of the assignment is the name of the member, as accessed by the syntax mentioned above. Because certain characters cannot be part of a literal string expression directly, they are instead identified by an escape sequence starting with a backslash (\). For example, the backslashes in "This string contains \"double quotes\"." indicate (to the compiler) that the inner pair of quotes are intended as an actual part of the string, rather than the default reading as a delimiter (endpoint) of the string itself. In C, string literals are surrounded by double quotes (") (e.g., "Hello world!") and are compiled to an array of the specified char values with an additional null terminating character (0-valued) code to mark the end of the string. This ensures that further attempts to dereference the pointer, on most systems, will crash the program.
If there are no parameters, the may be left empty or optionally be specified with the single word void. A continue not contained within a nested iteration statement is the same as goto cont. The identifier must be a label (followed by a colon) located in the current function. A missing second expression makes the while test always non-zero, creating a potentially infinite loop. In the if statement, if the in parentheses is nonzero (true), control passes to . If the else clause is present and the is zero (false), control will pass to .
If signed or unsigned is not specified explicitly, in most circumstances, signed is assumed. However, for historic reasons, plain char is a type distinct from both signed char and unsigned char. It may be a signed type or an unsigned type, depending on the compiler and the character set (C guarantees that members of the C basic character set have positive values). Also, bit field types specified as plain int may be signed or unsigned, depending on the compiler. POSIX, as well as SUS, specify a number of routines that should be available over and above those in the basic C standard library. The POSIX specification includes header files for, among other uses, multi-threading, networking, and regular expressions.
The book introduced the "Hello, World!" program, which prints only the text "hello, world", as an illustration of a minimal working C program. Since then, many texts have followed that convention for introducing a programming language. In cases where code must be compilable by either standard-conforming or K&R C-based compilers, the __STDC__ macro can be used to split the code into Standard and K&R sections to prevent the use on a K&R C-based compiler of features available only in Standard C. In 1978, Brian Kernighan and Dennis Ritchie published the first edition of The C Programming Language.[17] Known as K&R from the initials of its authors, the book served for many years as an informal specification of the language. As this was released in 1978, it is now also referred to as C78.[18] The second edition of the book[19] covers the later ANSI C standard, described below. At Version 4 Unix, released in November 1973, the Unix kernel was extensively re-implemented in C.[8] By this time, the C language had acquired some powerful features such as struct types.
Lyra Therapeutics Announces Inducement Grants Under Nasdaq Listing Rule 5635(c)(4) - Yahoo Finance
Lyra Therapeutics Announces Inducement Grants Under Nasdaq Listing Rule 5635(c)( .
Posted: Fri, 26 Apr 2024 20:51:00 GMT [source]
In C, all executable code is contained within subroutines (also called "functions", though not in the sense of functional programming). Function parameters are passed by value, although arrays are passed as pointers, i.e. the address of the first item in the array. Pass-by-reference is simulated in C by explicitly passing pointers to the thing being referenced.
Comments
Post a Comment