C programming language Wikipedia
Table Of Content

And are used as the body of a function or anywhere that a single statement is expected. The declaration-list declares variables to be used in that scope, and the statement-list are the actions to be performed. Brackets define their own scope, and variables defined inside those brackets will be automaticallydeallocated at the closing bracket. Declarations and statements can be freely intermixed within a compound statement (as in C++). Wide characters are most commonly either 2 bytes (using a 2-byte encoding such as UTF-16) or 4 bytes (usually UTF-32), but Standard C does not specify the width for wchar_t, leaving the choice to the implementor.
GCC 13 compiler collection backs C++ 23, Go 1.18
Tempest Reports Inducement Grant Under Nasdaq Listing Rule 5635(c)(4) - Yahoo Finance
Tempest Reports Inducement Grant Under Nasdaq Listing Rule 5635(c)( .
Posted: Fri, 26 Apr 2024 20:05:00 GMT [source]
Whether it references a local variable on the stack, or a register mapped into RAM, in the end, it’s all just data behind an address. And by that logic, if we can do something like some_function(®ular_variable) in C, i.e. pass a pointer as parameter to a function, we should be able to do the same with registers. On a system with a memory management unit, such as your average desktop computer, you will most certainly end up with a segmentation fault in the last line. The compiler complained because we wrote an integer into a variable that should be a pointer to an integer, and we should have listened.
White House urges developers to dump C and C++
C has also been widely used to implement end-user applications.[53] However, such applications can also be written in newer, higher-level languages. The "hello, world" example, which appeared in the first edition of K&R, has become the model for an introductory program in most programming textbooks. The program prints "hello, world" to the standard output, which is usually a terminal or screen display. Because of the language's grammar, a scalar initializer may be enclosed in any number of curly brace pairs.
Primitive data types
However, in early versions of C the bounds of the array must be known fixed values or else explicitly passed to any subroutine that requires them, and dynamically sized arrays of arrays cannot be accessed using double indexing. (A workaround for this was to allocate the array with an additional "row vector" of pointers to the columns.) C99 introduced "variable-length arrays" which address this issue. Array types in C are traditionally of a fixed, static size specified at compile time. The more recent C99 standard also allows a form of variable-length arrays.
C has some features, such as line-number preprocessor directives and optional superfluous commas at the end of initializer lists, that support compilation of generated code. However, some of C's shortcomings have prompted the development of other C-based languages specifically designed for use as intermediate languages, such as C--. Also, contemporary major compilers GCC and LLVM both feature an intermediate representation that is not C, and those compilers support front ends for many languages including C. Furthermore, in most expression contexts (a notable exception is as operand of sizeof), an expression of array type is automatically converted to a pointer to the array's first element.
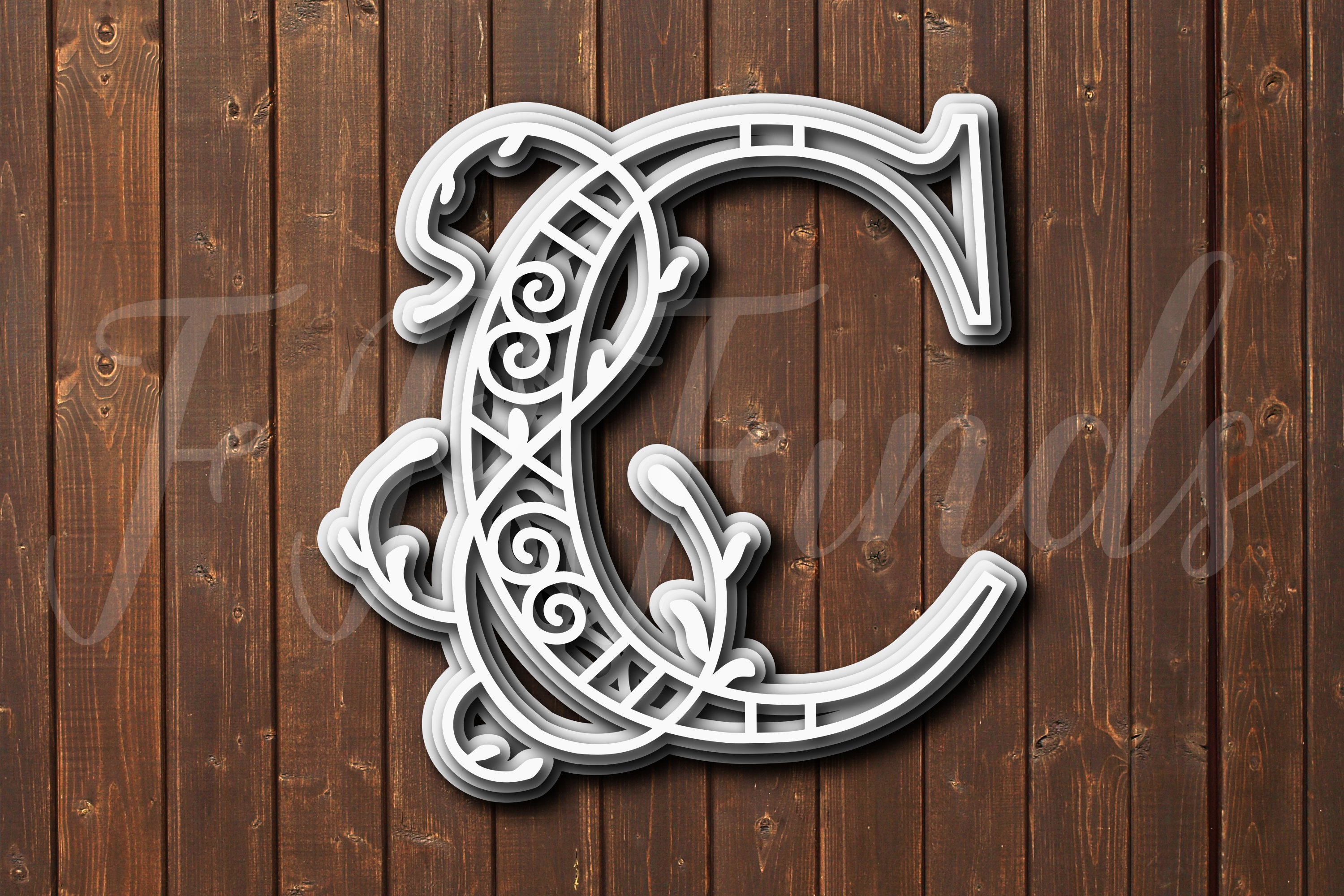
Assignment operators
In general though, setting pointers to NULL is good practice [according to whom? ] as it allows a programmer to NULL-check pointers prior to dereferencing, thus helping prevent crashes. Such array variables are allocated based on the value of an integer value at runtime upon entry to a block, and are deallocated at the end of the block.[1] As of C11 this feature is no longer required to be implemented by the compiler. The _Thread_local (thread_local in C++, since C23, and in earlier versions of C if the header is included) storage class specifier, introduced in C11, is used to declare a thread-local variable. The extern storage class specifier indicates that the storage for an object has been defined elsewhere. When used inside a block, it indicates that the storage has been defined by a declaration outside of that block.
Implementations
The now generally recommended method[note 3] of supporting international characters is through UTF-8, which is stored in char arrays, and can be written directly in the source code if using a UTF-8 editor, because UTF-8 is a direct ASCII extension. A multidimensional array should not be confused with an array of pointers to arrays (also known as an Iliffe vector or sometimes an array of arrays). The former is always rectangular (all subarrays must be the same size), and occupies a contiguous region of memory. The latter is a one-dimensional array of pointers, each of which may point to the first element of a subarray in a different place in memory, and the sub-arrays do not have to be the same size. The type qualifier const indicates that a value does not change once it has been initialized. Attempting to modify a const qualified value yields undefined behavior, so some C compilers store them in rodata or (for embedded systems) in read-only memory (ROM).
POSIX standard library
However, such an object can be assigned any values in the range of their compatible type, and enum constants can be used anywhere an integer is expected. For this reason, enum values are often used in place of preprocessor #define directives to create named constants. Such constants are generally safer to use than macros, since they reside within a specific identifier namespace. The char type is distinct from both signed char and unsigned char, but is guaranteed to have the same representation as one of them. The _Bool and long long types are standardized since 1999, and may not be supported by older C compilers. Type _Bool is usually accessed via the typedef name bool defined by the standard header stdbool.h.
Data types
Part of the resulting standard was a set of software libraries called the ANSI C standard library. The original C language provided no built-in functions such as I/O operations, unlike traditional languages such as COBOL and Fortran.[citation needed] Over time, user communities of C shared ideas and implementations of what is now called C standard libraries. Many of these ideas were incorporated eventually into the definition of the standardized C language. On Unix-like systems, the authoritative documentation of the API is provided in the form of man pages.
The original C standard specified only minimal functions for operating with wide character strings; in 1995 the standard was modified to include much more extensive support, comparable to that for char strings. The relevant functions are mostly named after their char equivalents, with the addition of a "w" or the replacement of "str" with "wcs"; they are specified in , with containing wide-character classification and mapping functions. Compiled applications written in C are either statically linked with a C library, or linked to a dynamic version of the library that is shipped with these applications, rather than relied upon to be present on the targeted systems. Functions in a compiler's C library are not regarded as interfaces to Microsoft Windows. C is sometimes used as an intermediate language by implementations of other languages. This approach may be used for portability or convenience; by using C as an intermediate language, additional machine-specific code generators are not necessary.
Pointers — you either love them, or you haven’t fully understood them yet. But before you storm off to the comment section now, pointers are indeed a polarizing subject and are both C’s biggest strength, and its major source of problems. The internet and libraries are full of tutorials and books telling about pointers, and you can randomly pick pretty much any one of them and you’ll be good to go. However, while the basic principles of pointers are rather simple in theory, it can be challenging to fully wrap your head around their purpose and exploit their true potential. Most of the operators available in C and C++ are also available in other C-family languages such as C#, D, Java, Perl, and PHP with the same precedence, associativity, and semantics. During the late 1970s and 1980s, versions of C were implemented for a wide variety of mainframe computers, minicomputers, and microcomputers, including the IBM PC, as its popularity began to increase significantly.
Newline indicates the end of a text line; it need not correspond to an actual single character, although for convenience C treats it as one. ANSI, like other national standards bodies, no longer develops the C standard independently, but defers to the international C standard, maintained by the working group ISO/IEC JTC1/SC22/WG14. National adoption of an update to the international standard typically occurs within a year of ISO publication.
Moreover, in C++ (and later versions of C) equality operations, with the exception of the three-way comparison operator, yield bool type values which are conceptually a single bit (1 or 0) and as such do not properly belong in "bitwise" operations. The following is a table that lists the precedence and associativity of all the operators in the C and C++ languages. Descending precedence refers to the priority of the grouping of operators and operands. Considering an expression, an operator which is listed on some row will be grouped prior to any operator that is listed on a row further below it. Operators that are in the same cell (there may be several rows of operators listed in a cell) are grouped with the same precedence, in the given direction. For the purposes of these tables, a, b, and c represent valid values (literals, values from variables, or return value), object names, or lvalues, as appropriate.
For example, a comparison of signed and unsigned integers of equal width requires a conversion of the signed value to unsigned. In 2008, the C Standards Committee published a technical report extending the C language[26] to address these issues by providing a common standard for all implementations to adhere to. It includes a number of features not available in normal C, such as fixed-point arithmetic, named address spaces, and basic I/O hardware addressing. While individual strings are arrays of contiguous characters, there is no guarantee that the strings are stored as a contiguous group. The C standard defines return values 0 and EXIT_SUCCESS as indicating success and EXIT_FAILURE as indicating failure. Other return values have implementation-defined meanings; for example, under Linux a program killed by a signal yields a return code of the numerical value of the signal plus 128.
Microsoft Windows generally uses UTF-16, thus the above string would be 26 bytes long for a Microsoft compiler; the Unix world prefers UTF-32, thus compilers such as GCC would generate a 52-byte string. A 2-byte wide wchar_t suffers the same limitation as char, in that certain characters (those outside the BMP) cannot be represented in a single wchar_t; but must be represented using surrogate pairs. Note that storage specifiers apply only to functions and objects; other things such as type and enum declarations are private to the compilation unit in which they appear. In addition to the standard integer types, there may be other "extended" integer types, which can be used for typedefs in standard headers.
A successor to the programming language B, C was originally developed at Bell Labs by Ritchie between 1972 and 1973 to construct utilities running on Unix. It was applied to re-implementing the kernel of the Unix operating system.[8] During the 1980s, C gradually gained popularity. It has become one of the most widely used programming languages,[9][10] with C compilers available for practically all modern computer architectures and operating systems. An implementation of C providing all of the standard library functions is called a hosted implementation. Programs written for hosted implementations are required to define a special function called main, which is the first function called when a program begins executing.
Comments
Post a Comment